Let us say you want to create a simple form to persist some coordinates with metadata using Streamlit.
In order to make things easier you want to allow the analysts to choose the position using a map.
There is a great plugin called streamlit-folium which you can use to achieve that.
To use it just run `pip install streamlit-folium`.
The example below presents how you can quickly retrieve the coordinates and submit them using standard Streamlit form.
import streamlit as st
from streamlit_folium import st_folium
import folium
DEFAULT_LATITUDE = 51.
DEFAULT_LONGITUDE = 3.
m = folium.Map(location=[DEFAULT_LATITUDE, DEFAULT_LONGITUDE], zoom_start=10)
# The code below will be responsible for displaying
# the popup with the latitude and longitude shown
m.add_child(folium.LatLngPopup())
f_map = st_folium(m, width=725)
selected_latitude = DEFAULT_LATITUDE
selected_longitude = DEFAULT_LONGITUDE
if f_map.get("last_clicked"):
selected_latitude = f_map["last_clicked"]["lat"]
selected_longitude = f_map["last_clicked"]["lng"]
form = st.form("Position entry form")
submit = form.form_submit_button()
if submit:
if selected_latitude == DEFAULT_LATITUDE and selected_longitude == DEFAULT_LONGITUDE:
st.warning("Selected position has default values!")
st.success(f"Stored position: {selected_latitude}, {selected_longitude}")
This is how it should look like if you run this example.
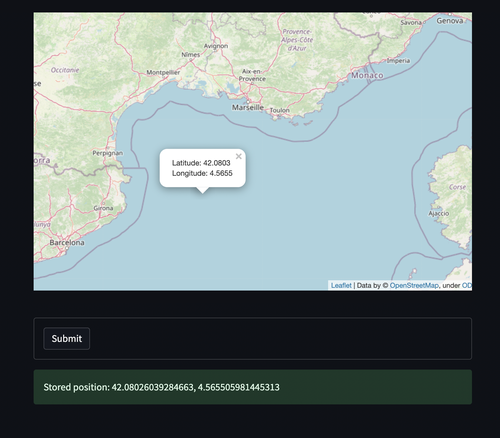
Streamlit is a great framework to use for building minimal viable products for internal usage.
You can quickly get 80% of the work done and validate your ideas.